Rx is a generic abstraction of computation expressed through Observable<Element>
interface, which lets you broadcast and subscribe to values and other events from an Observable
stream.
RxSwift is the Swift-specific implementation of the Reactive Extensions standard.
While this version aims to stay true to the original spirit and naming conventions of Rx, this project also aims to provide a true Swift-first API for Rx APIs.
Cross platform documentation can be found on ReactiveX.io.
Like other Rx implementations, RxSwift's intention is to enable easy composition of asynchronous operations and streams of data in the form of Observable
objects and a suite of methods to transform and compose these pieces of asynchronous work.
KVO observation, async operations, UI Events and other streams of data are all unified under abstraction of sequence. This is the reason why Rx is so simple, elegant and powerful.
- why use rx?
- the basics, getting started with RxSwift
- traits - what are
Single
,Completable
,Maybe
,Driver
, andControlProperty
... and why do they exist? - testing
- tips and common errors
- debugging
- the math behind Rx
- what are hot and cold observable sequences?
- Integrate RxSwift/RxCocoa with my app. Installation Guide
- with the example app. Running Example App
- with operators in playgrounds. Playgrounds
- All of this is great, but it would be nice to talk with other people using RxSwift and exchange experiences.
Join Slack Channel - Report a problem using the library. Open an Issue With Bug Template
- Request a new feature. Open an Issue With Feature Request Template
- Help out Check out contribution guide
RxSwift is as compositional as the asynchronous work it drives. The core unit is RxSwift itself, while other dependencies can be added for UI Work, testing, and more.
It comprises five separate components depending on each other in the following way:
┌──────────────┐ ┌──────────────┐
│ RxCocoa ├────▶ RxRelay │
└───────┬──────┘ └──────┬───────┘
│ │
┌───────▼──────────────────▼───────┐
│ RxSwift │
└───────▲──────────────────▲───────┘
│ │
┌───────┴──────┐ ┌──────┴───────┐
│ RxTest │ │ RxBlocking │
└──────────────┘ └──────────────┘
- RxSwift: The core of RxSwift, providing the Rx standard as (mostly) defined by ReactiveX. It has no other dependencies.
- RxCocoa: Provides Cocoa-specific capabilities for general iOS/macOS/watchOS & tvOS app development, such as Shared Sequences, Traits, and much more. It depends on both
RxSwift
andRxRelay
. - RxRelay: Provides
PublishRelay
,BehaviorRelay
andReplayRelay
, three simple wrappers around Subjects. It depends onRxSwift
. - RxTest and RxBlocking: Provides testing capabilities for Rx-based systems. It depends on
RxSwift
.
RxSwift doesn't contain any external dependencies.
These are currently the supported installation options:
Open Rx.xcworkspace, choose RxExample
and hit run. This method will build everything and run the sample app
# Podfile
use_frameworks!
target 'YOUR_TARGET_NAME' do
pod 'RxSwift', '6.7.0'
pod 'RxCocoa', '6.7.0'
end
# RxTest and RxBlocking make the most sense in the context of unit/integration tests
target 'YOUR_TESTING_TARGET' do
pod 'RxBlocking', '6.7.0'
pod 'RxTest', '6.7.0'
end
Replace YOUR_TARGET_NAME
and then, in the Podfile
directory, type:
$ pod install
Each release starting with RxSwift 6 includes *.xcframework
framework binaries.
Simply drag the needed framework binaries to your Frameworks, Libraries, and Embedded Content section under your target's General tab.
Tip
You may verify the identity of the binaries by comparing against the following fingerprint in Xcode 15+:
BD 80 2E 79 4C 8A BD DA 4C 3F 5D 92 B3 E4 C4 FB FA E4 73 44 10 B9 AD 73 44 2E F1 CE B0 27 61 40
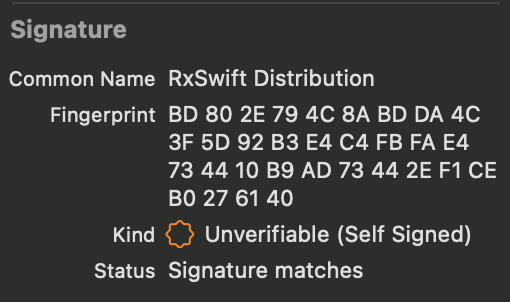
Add this to Cartfile
github "ReactiveX/RxSwift" "6.7.0"
$ carthage update
Carthage defaults to building RxSwift as a Dynamic Library.
If you wish to build RxSwift as a Static Library using Carthage you may use the script below to manually modify the framework type before building with Carthage:
carthage update RxSwift --platform iOS --no-build
sed -i -e 's/MACH_O_TYPE = mh_dylib/MACH_O_TYPE = staticlib/g' Carthage/Checkouts/RxSwift/Rx.xcodeproj/project.pbxproj
carthage build RxSwift --platform iOS
Note: There is a critical cross-dependency bug affecting many projects including RxSwift in Swift Package Manager. We've filed a bug (SR-12303) in early 2020 but have no answer yet. Your mileage may vary. A partial workaround can be found here.
Create a Package.swift
file.
// swift-tools-version:5.0
import PackageDescription
let package = Package(
name: "RxProject",
dependencies: [
.package(url: "https://github.com/ReactiveX/RxSwift.git", .upToNextMajor(from: "6.0.0"))
],
targets: [
.target(name: "RxProject", dependencies: ["RxSwift", .product(name: "RxCocoa", package: "RxSwift")]),
]
)
$ swift build
To build or test a module with RxTest dependency, set TEST=1
.
$ TEST=1 swift test
- Add RxSwift as a submodule
$ git submodule add [email protected]:ReactiveX/RxSwift.git
- Drag
Rx.xcodeproj
into Project Navigator - Go to
Project > Targets > Build Phases > Link Binary With Libraries
, click+
and selectRxSwift
,RxCocoa
andRxRelay
targets
- http://reactivex.io/
- Reactive Extensions GitHub (GitHub)
- RxSwift RayWenderlich.com Book
- RxSwift: Debunking the myth of hard (YouTube)
- Boxue.io RxSwift Online Course (Chinese 🇨🇳)
- Expert to Expert: Brian Beckman and Erik Meijer - Inside the .NET Reactive Framework (Rx) (video)
- Reactive Programming Overview (Jafar Husain from Netflix)
- Subject/Observer is Dual to Iterator (paper)
- Rx standard sequence operators visualized (visualization tool)
- Haskell