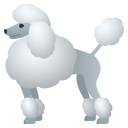
Simple and complete Preact DOM testing utilities that encourage good testing practices.
Inspired completely by react-testing-library
You want to write tests for your Preact components so that they avoid including implementation details, and are maintainable in the long run.
The Preact Testing Library is a very lightweight solution for testing Preact components. It provides light utility functions on top of preact/test-utils, in a way that encourages better testing practices. Its primary guiding principle is:
The more your tests resemble the way your software is used, the more confidence they can give you.
This module is distributed via npm which is bundled with node and should be installed
as one of your project's devDependencies
:
npm install --save-dev @testing-library/preact
This library has peerDependencies
listings for preact >= 10
.
๐ก You may also be interested in installing @testing-library/jest-dom
so you can use
the custom jest matchers.
๐ This library supports Preact X (10.x). It takes advantage of the act
test utility in
preact/test-utils
to enable both Preact Hook and Class components to be easily tested.
๐ If you're looking for a solution for Preact 8.x then install preact-testing-library
.
See the docs over at the Testing Library website.
Looking to contribute? Look for the Good First Issue label.
Please file an issue for bugs, missing documentation, or unexpected behavior.
Please file an issue to suggest new features. Vote on feature requests by adding a ๐. This helps maintainers prioritize what to work on.
For questions related to using the library, please visit a support community instead of filing an issue on GitHub.
Thanks goes to these people (emoji key):
Kent C. Dodds ๐ป ๐ |
Ants Martian ๐ป ๐ |
Rahim Alwer ๐ป ๐ |
This project follows the all-contributors specification. Contributions of any kind welcome!