
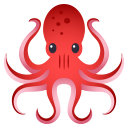
ESLint plugin to follow best practices and anticipate common mistakes when writing tests with Testing Library
You'll first need to install ESLint:
$ npm install --save-dev eslint
# or
$ yarn add --dev eslint
Next, install eslint-plugin-testing-library
:
$ npm install --save-dev eslint-plugin-testing-library
# or
$ yarn add --dev eslint-plugin-testing-library
Note: If you installed ESLint globally (using the -g
flag) then you must also install eslint-plugin-testing-library
globally.
You can find detailed guides for migrating eslint-plugin-testing-library
in the migration guide docs:
Add testing-library
to the plugins section of your .eslintrc.js
configuration file. You can omit the eslint-plugin-
prefix:
module.exports = {
plugins: ['testing-library'],
};
Then configure the rules you want to use within rules
property of your .eslintrc
:
module.exports = {
rules: {
'testing-library/await-async-queries': 'error',
'testing-library/no-await-sync-queries': 'error',
'testing-library/no-debugging-utils': 'warn',
'testing-library/no-dom-import': 'off',
},
};
With the default setup mentioned before, eslint-plugin-testing-library
will be run against your whole codebase. If you want to run this plugin only against your tests files, you have the following options:
One way of restricting ESLint config by file patterns is by using ESLint overrides
.
Assuming you are using the same pattern for your test files as Jest by default, the following config would run eslint-plugin-testing-library
only against your test files:
// .eslintrc.js
module.exports = {
// 1) Here we have our usual config which applies to the whole project, so we don't put testing-library preset here.
extends: ['airbnb', 'plugin:prettier/recommended'],
// 2) We load other plugins than eslint-plugin-testing-library globally if we want to.
plugins: ['react-hooks'],
overrides: [
{
// 3) Now we enable eslint-plugin-testing-library rules or preset only for matching testing files!
files: ['**/__tests__/**/*.[jt]s?(x)', '**/?(*.)+(spec|test).[jt]s?(x)'],
extends: ['plugin:testing-library/react'],
},
],
};
Another approach for customizing ESLint config by paths is through ESLint Cascading and Hierarchy. This is useful if all your tests are placed under the same folder, so you can place there another .eslintrc
where you enable eslint-plugin-testing-library
for applying it only to the files under such folder, rather than enabling it on your global .eslintrc
which would apply to your whole project.
This plugin exports several recommended configurations that enforce good practices for specific Testing Library packages.
You can find more info about enabled rules in the Supported Rules section, under the Configurations
column.
Since each one of these configurations is aimed at a particular Testing Library package, they are not extendable between them, so you should use only one of them at once per .eslintrc
file. For example, if you want to enable recommended configuration for React, you don't need to combine it somehow with DOM one:
// ❌ Don't do this
module.exports = {
extends: ['plugin:testing-library/dom', 'plugin:testing-library/react'],
};
// ✅ Just do this instead
module.exports = {
extends: ['plugin:testing-library/react'],
};
Enforces recommended rules for DOM Testing Library.
To enable this configuration use the extends
property in your
.eslintrc.js
config file:
module.exports = {
extends: ['plugin:testing-library/dom'],
};
Enforces recommended rules for Angular Testing Library.
To enable this configuration use the extends
property in your
.eslintrc.js
config file:
module.exports = {
extends: ['plugin:testing-library/angular'],
};
Enforces recommended rules for React Testing Library.
To enable this configuration use the extends
property in your
.eslintrc.js
config file:
module.exports = {
extends: ['plugin:testing-library/react'],
};
Enforces recommended rules for Vue Testing Library.
To enable this configuration use the extends
property in your
.eslintrc.js
config file:
module.exports = {
extends: ['plugin:testing-library/vue'],
};
Enforces recommended rules for Marko Testing Library.
To enable this configuration use the extends
property in your
.eslintrc.js
config file:
module.exports = {
extends: ['plugin:testing-library/marko'],
};
Remember that all rules from this plugin are prefixed by
"testing-library/"
💼 Configurations enabled in.
🔧 Automatically fixable by the --fix
CLI option.
Name | Description | 💼 | 🔧 | |
---|---|---|---|---|
await-async-events | Enforce promises from async event methods are handled | 🔧 | ||
await-async-queries | Enforce promises from async queries to be handled | |||
await-async-utils | Enforce promises from async utils to be awaited properly | |||
consistent-data-testid | Ensures consistent usage of data-testid |
|||
no-await-sync-events | Disallow unnecessary await for sync events |
|||
no-await-sync-queries | Disallow unnecessary await for sync queries |
|||
no-container | Disallow the use of container methods |
|||
no-debugging-utils | Disallow the use of debugging utilities like debug |
|||
no-dom-import | Disallow importing from DOM Testing Library | 🔧 | ||
no-global-regexp-flag-in-query | Disallow the use of the global RegExp flag (/g) in queries | 🔧 | ||
no-manual-cleanup | Disallow the use of cleanup |
|||
no-node-access | Disallow direct Node access | |||
no-promise-in-fire-event | Disallow the use of promises passed to a fireEvent method |
|||
no-render-in-lifecycle | Disallow the use of render in testing frameworks setup functions |
|||
no-unnecessary-act | Disallow wrapping Testing Library utils or empty callbacks in act |
|||
no-wait-for-multiple-assertions | Disallow the use of multiple expect calls inside waitFor |
|||
no-wait-for-side-effects | Disallow the use of side effects in waitFor |
|||
no-wait-for-snapshot | Ensures no snapshot is generated inside of a waitFor call |
|||
prefer-explicit-assert | Suggest using explicit assertions rather than standalone queries | |||
prefer-find-by | Suggest using find(All)By* query instead of waitFor + get(All)By* to wait for elements |
🔧 | ||
prefer-implicit-assert | Suggest using implicit assertions for getBy* & findBy* queries | |||
prefer-presence-queries | Ensure appropriate get* /query* queries are used with their respective matchers |
|||
prefer-query-by-disappearance | Suggest using queryBy* queries when waiting for disappearance |
|||
prefer-query-matchers | Ensure the configured get* /query* query is used with the corresponding matchers |
|||
prefer-screen-queries | Suggest using screen while querying |
|||
prefer-user-event | Suggest using userEvent over fireEvent for simulating user interactions |
|||
render-result-naming-convention | Enforce a valid naming for return value from render |
In v4 this plugin introduced a new feature called "Aggressive Reporting", which intends to detect Testing Library utils usages even if they don't come directly from a Testing Library package (i.e. using a custom utility file to re-export everything from Testing Library). You can read more about this feature here.
If you are looking to restricting or switching off this feature, please refer to the Shared Settings section to do so.
There are some configuration options available that will be shared across all the plugin rules. This is achieved using ESLint Shared Settings. These Shared Settings are meant to be used if you need to restrict or switch off the Aggressive Reporting, which is an out of the box advanced feature to lint Testing Library usages in a simpler way for most of the users. So please before configuring any of these settings, read more about the advantages of eslint-plugin-testing-library
Aggressive Reporting feature, and how it's affected by these settings.
If you are sure about configuring the settings, these are the options available:
The name of your custom utility file from where you re-export everything from the Testing Library package, or "off"
to switch related Aggressive Reporting mechanism off. Relates to Aggressive Imports Reporting.
// .eslintrc.js
module.exports = {
settings: {
'testing-library/utils-module': 'my-custom-test-utility-file',
},
};
You can find more details about the utils-module
setting here.
A list of function names that are valid as Testing Library custom renders, or "off"
to switch related Aggressive Reporting mechanism off. Relates to Aggressive Renders Reporting.
// .eslintrc.js
module.exports = {
settings: {
'testing-library/custom-renders': ['display', 'renderWithProviders'],
},
};
You can find more details about the custom-renders
setting here.
A list of query names/patterns that are valid as Testing Library custom queries, or "off"
to switch related Aggressive Reporting mechanism off. Relates to Aggressive Reporting - Queries
// .eslintrc.js
module.exports = {
settings: {
'testing-library/custom-queries': ['ByIcon', 'getByComplexText'],
},
};
You can find more details about the custom-queries
setting here.
Since each Shared Setting is related to one Aggressive Reporting mechanism, and they accept "off"
to opt out of that mechanism, you can switch the entire feature off by doing:
// .eslintrc.js
module.exports = {
settings: {
'testing-library/utils-module': 'off',
'testing-library/custom-renders': 'off',
'testing-library/custom-queries': 'off',
},
};
If you find ESLint errors related to eslint-plugin-testing-library
in files other than testing, this could be caused by Aggressive Reporting.
You can avoid this by:
- running
eslint-plugin-testing-library
only against testing files - limiting the scope of Aggressive Reporting through Shared Settings
- switching Aggressive Reporting feature off
If you think the error you are getting is not related to this at all, please fill a new issue with as many details as possible.
If you are getting false positive ESLint errors in your testing files, this could be caused by Aggressive Reporting.
You can avoid this by:
- limiting the scope of Aggressive Reporting through Shared Settings
- switching Aggressive Reporting feature off
If you think the error you are getting is not related to this at all, please fill a new issue with as many details as possible.
Thanks goes to these wonderful people (emoji key):
This project follows the all-contributors specification. Contributions of any kind welcome!