javascript related stuff
Please donate if you are using this repo
Note: If you found this project helpful please give this repo a star.
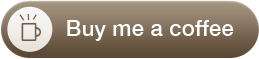
- Load js and css files
- Find the largest number contained in a JavaScript array
- Find the smallest number contained in a JavaScript array
- Angular 2 cli
- Angular 2 Mobile Toolkit
- html2canvas: Screenshots with JavaScript
- GPU and Web UI Performance: Building an Endless 60fps Scroller
- Mapzen: is an open, sustainable, and accessible mapping platform. Our tools let you display, search, and navigate your world
- Simple Statistics: is a JavaScript library that implements statistical methods
- regression.js: linear, exponential, logarithmic, power and polynomial trends
- Live Code Session - Supercharged
- hello.js: A client-side JavaScript SDK for authenticating with OAuth
- Thorin.js: is the Node.js framework
- Check if element is visible after scrolling
- Propeller.js: JavaScript library to rotate elements with mouse or touch gestures
- Stickybits is a lightweight alternative to position: sticky polyfills. It works perfectly for things like sticky headers.
- Sortable
- Interact.js: drag and drop, resizing and multi-touch gestures with inertia and snapping
- Gridster.js
- Bowser: A Browser detector
- Idle Logout
- Shuffle: Categorize, sort, and filter a responsive grid of items
- Filterizr: is a jQuery plugin that sorts, shuffles and applies stunning filters over responsive galleries using CSS3 transitions and custom CSS effects
- JavaScript library of crypto standards
- ripplet.js: Fully controllable vanilla-js material design ripple effect generator
- js-effect-ripple: Material Design Ripple effect in pure JS & CSS
- Ocrad.js Optical Character Recognition in JS
- Tesseract.js is a pure Javascript port of the popular Tesseract OCR engine
function loadScript(url, callback)
{
// Adding the script tag to the head as suggested before
var head = document.getElementsByTagName('head')[0];
var script = document.createElement('script');
script.type = 'text/javascript';
script.src = url;
// Then bind the event to the callback function.
// There are several events for cross browser compatibility.
script.onreadystatechange = callback;
script.onload = callback;
// Fire the loading
head.appendChild(script);
}
var myPrettyCode = function() {
// Here, do what ever you want
};
loadScript("my_lovely_script.js", myPrettyCode);
function loadjscssfile(filename, filetype) {
if (filetype == "js") { //if filename is a external JavaScript file
// alert('called');
var fileref = document.createElement('script')
fileref.setAttribute("type", "text/javascript")
fileref.setAttribute("src", filename)
alert('called');
}
else if (filetype == "css") { //if filename is an external CSS file
var fileref = document.createElement("link")
fileref.setAttribute("rel", "stylesheet")
fileref.setAttribute("type", "text/css")
fileref.setAttribute("href", filename)
}
if (typeof fileref != "undefined")
document.getElementsByTagName("head")[0].appendChild(fileref)
}
var array = [267, 306, 108];
Math.max.apply(Math, array);
Result: 306
var array = [267, 306, 108];
Math.min.apply(Math, array);
Result: 108
GPU and Web UI Performance: Building an Endless 60fps Scroller
Simple Statistics is a JavaScript library that implements statistical methods
YouTube: Supercharged with Paul Lewis & Surma
UI Elements: A collection of UI element samples written with vanilla web platform features
A client-side JavaScript SDK for authenticating with OAuth2 (and OAuth1 with a oauth proxy) web services and querying their REST APIs.
Thorin.js is the Node.js framework that you can use to easily:
- Boot up a pre-configured web-server, sanitize and validate all your input
- Render static HTML files using a templating engine, compile your less & sass styles
- Integrate centralized logging with loglet.io
- Use secure sessions in your requests, stored in redis, MySQL or file system
- Use already written components for some wide-spread services (Redis, ElasticSearch, Sequelize)
- Use a centralized dispatcher to handle your redux actions via HTTP or WebSockets
- Schedule recurring tasks that may use a redis queue to process events
- Extend the core functionality with use-case specific plugins
- Integrate with sconfig.io's discovery system for microservice discovery & authorization
- And much much more.
function isScrolledIntoView(el) {
var elemTop = el.getBoundingClientRect().top;
var elemBottom = el.getBoundingClientRect().bottom;
var isVisible = (elemTop >= 0) && (elemBottom <= window.innerHeight);
return isVisible;
}
Propeller.js: JavaScript library to rotate elements with mouse or touch gestures. Supports inertia and stepwise rotation. Optimized for better performance
Stickybits is a lightweight alternative to position: sticky polyfills. It works perfectly for things like sticky headers.
function idleLogout() {
var t;
window.onload = resetTimer;
window.onmousemove = resetTimer;
window.onmousedown = resetTimer; // catches touchscreen presses
window.onclick = resetTimer; // catches touchpad clicks
window.onscroll = resetTimer; // catches scrolling with arrow keys
window.onkeypress = resetTimer;
function logout() {
window.location.href = 'logout.php';
}
function resetTimer() {
clearTimeout(t);
t = setTimeout(logout, 10000); // time is in milliseconds
}
}
idleLogout();